Java is a powerful and versatile programming language that allows developers to create robust and efficient applications. One of the foundational concepts in Java is the interface. In this post, we'll explain what interfaces are, why they are essential, and how practical they are in real-world applications.
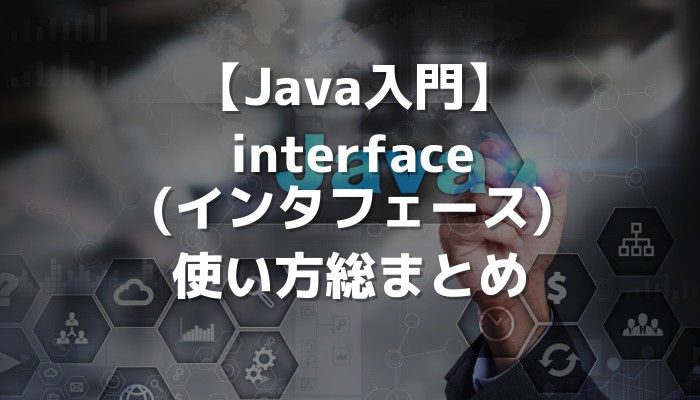
What are Java Interfaces?
In Java, an interface is a blueprint for a class that defines the structure of a set of methods without providing their implementation. In other words, interfaces allow you to define the "what" (method signatures) without specifying the "how" (method implementation).
Why Use Interfaces?
Interfaces are crucial for several reasons:
1. Abstraction: Interfaces enable developers to separate the functionality of a method from its implementation. By doing this, you can change the underlying implementation without affecting the code that depends on the interface.
2. Code reusability: Interfaces promote code reusability by allowing multiple classes to implement the same interface. This way, you can use a common set of methods in various situations without duplicating code.
3. Flexibility: With interfaces, you can easily swap out different implementations of the same functionality, which makes your code more adaptable to changes in requirements.
Practicality of Interfaces
Let's take a look at a practical example to demonstrate the usefulness of interfaces. Imagine you're building a drawing application that supports various shapes like circles, squares, and triangles. To ensure consistency, you want each shape to have a `draw()` method.
interface Drawable {
void draw();
}
By defining a `Drawable` interface with a `draw()` method, you set up a contract that each shape class must follow. Now, you can create classes for each shape that implements the `Drawable` interface:
class Circle implements Drawable {
@Override
void draw() {
System.out.println("Drawing a circle");
}
}
class Square implements Drawable {
@Override
void draw() {
System.out.println("Drawing a square");
}
}
With these classes in place, you can easily add new shapes or change the drawing logic without affecting the rest of your application. The interface ensures that each shape class provides a consistent method for drawing, making your code more maintainable and easy to understand.
Exercises
To help you practice the concept of interfaces and implementation, try these two exercises:
Create an interface called Comparable that has a single method int compare(Comparable other). Implement this interface in two classes, Person and Car, where the compare() method compares two instances of the respective classes based on their ages (for Person) and manufacturing years (for Car).
Create an interface called Printable with a method void print(). Implement this interface in two classes, Book and Invoice. The print() method should display the title and author of the Book and the item details and total cost of the Invoice.
Conclusion
Java interfaces play a crucial role in promoting abstraction, code reusability, and flexibility in your applications. By understanding and using interfaces effectively, you can create cleaner, more adaptable code that's easier to maintain and modify as your project requirements evolve. Start leveraging the power of interfaces in Java today and take your programming skills to new heights!
Answers:
Exercise 1:
interface Comparable {
int compare(Comparable other);
}
class Person implements Comparable {
int age;
@Override
int compare(Comparable other) {
Person otherPerson = (Person) other;
return Integer.compare(this.age, otherPerson.age);
}
}
class Car implements Comparable {
int manufacturingYear;
@Override
int compare(Comparable other) {
Car otherCar = (Car) other;
return Integer.compare(this.manufacturingYear, otherCar.manufacturingYear);
}
}
Exercise 2:
interface Printable {
void print();
}
class Book implements Printable {
String title;
String author;
@Override
void print() {
System.out.println("Title: " + title + ", Author: " + author);
}
}
class Invoice implements Printable {
String itemDetails;
double totalCost;
@Override
void print() {
System.out.println("Item Details: " + itemDetails + ", Total Cost: " + totalCost);
}
}
Comments