Understanding Functions in Swift and Their Relation to Transformers
- Chris Wong
- May 30, 2023
- 3 min read
Updated: Jul 1, 2023
In this post, we'll explore functions in Swift, a powerful feature that allows developers to write reusable and modular code. We'll also draw parallels between Swift functions and Transformers, a type of deep learning model, to help you understand the importance of functions in programming.
Table of Contents
1. [Introduction to Functions in Swift](#introduction)
2. [Defining and Calling Functions](#defining-calling)
3. [Function Parameters and Return Types](#parameters-return)
4. [External and Local Parameter Names](#external-local)
5. [Variadic, In-Out, and Default Parameter Values](#advanced-parameters)
6. [Functions as First-Class Citizens](#first-class)
7. [Closures](#closures)
8. [Functions in Transformers](#functions-transformers)
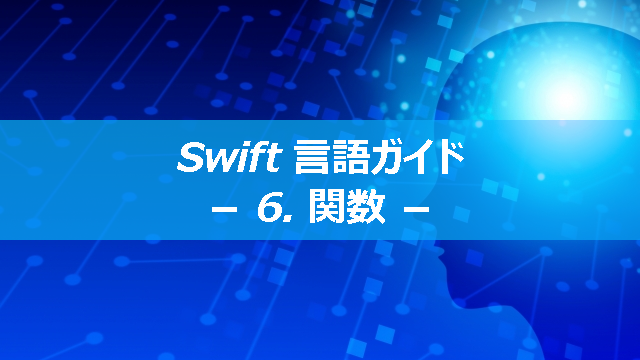
1. Introduction to Functions in Swift
Functions are self-contained blocks of code that perform a specific task. By encapsulating a piece of functionality within a function, you can easily reuse and maintain your code. Swift provides a variety of features to make functions flexible, readable, and easy to use.
2. Defining and Calling Functions
A function is declared using the `func` keyword, followed by the function name, a pair of parentheses, and a pair of braces that enclose the function's body. The function name should be descriptive and follow Swift's camelCase convention.
Here's a simple example of a function that prints "Hello, World!":
func printHelloWorld() {
print("Hello, World!")
}
// Calling the function
printHelloWorld()
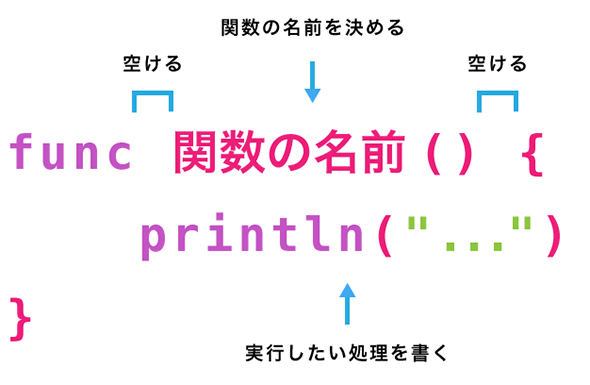
3. Function Parameters and Return Types
Functions can take input, known as parameters, and return output, known as the return type. Function parameters are declared within the parentheses after the function name, and the return type is declared after the `->` operator.
Example of a function with parameters and a return type:
func greet(name: String) -> String {
return "Hello, \(name)!"
}
let greeting = greet(name: "Alice")
print(greeting) // Output: Hello, Alice!
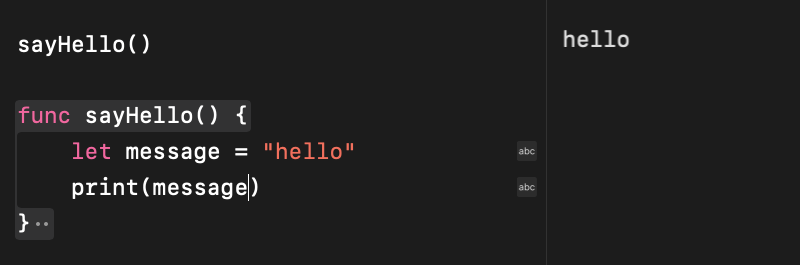
4. External and Local Parameter Names
Swift allows you to provide an external name for each parameter, making function calls more readable. The external name is written before the local name, separated by a space.
func greet(who name: String) -> String {
return "Hello, \(name)!"
}
let greeting = greet(who: "Bob")
print(greeting) // Output: Hello, Bob!
5. Variadic, In-Out, and Default Parameter Values
Swift functions support variadic parameters, allowing you to accept a variable number of arguments of the same type. You can also use in-out parameters to modify the value of a variable that was passed into the function. Default parameter values provide a default value when a parameter is not specified.
func sum(numbers: Int...) -> Int {
var total = 0
for number in numbers {
total += number
}
return total
}
print(sum(numbers: 1, 2, 3, 4)) // Output: 10
func increment(value: inout Int) {
value += 1
}
var number = 42
increment(value: &number)
print(number) // Output: 43
6. Functions as First-Class Citizens
In Swift, functions are first-class citizens, meaning you can use them as variables, pass them as arguments, or return them from other functions.
func square(_ x: Int) -> Int {
return x * x
}
func cube(_ x: Int) -> Int {
return x * x * x
}
func apply(operation: (Int) -> Int, to value: Int) -> Int {
return operation(value)
}
print(apply(operation: square, to: 3)) // Output: 9
print(apply(operation: cube, to: 3)) // Output: 27
7. Closures
Closures are self-contained, anonymous functions that capture and store references to variables and constants from the surrounding context. They can be used as arguments or return values in other functions.
let numbers = [1, 5, 2, 8, 3]
let sortedNumbers = numbers.sorted { (a: Int, b: Int) -> Bool in
return a < b
}
print(sortedNumbers) // Output: [1, 2, 3, 5, 8]
8. Functions in Transformers
Transformers are a type of deep learning model that has gained significant popularity due to their effectiveness in natural language processing tasks. They consist of layers and components that perform specific operations, such as self-attention, feed-forward networks, and normalization.
While Transformers are not written in Swift, understanding Swift functions can help you better comprehend the modularity and organization of Transformer architecture. Each component in a Transformer can be thought of as a function, taking inputs, processing them, and producing outputs.
For example, the self-attention mechanism in a Transformer can be seen as a function that takes a set of input tokens, calculates attention scores, and produces a new representation of the input tokens. Similarly, the feed-forward networks can be viewed as functions that further process these representations.
By breaking down complex models like Transformers into smaller, reusable components, developers and researchers can experiment with different configurations, improve the model's performance, and gain a deeper understanding of the underlying techniques.
In summary, understanding functions in Swift not only helps you write better code in Swift but also provides a foundation for understanding more complex structures, such as Transformer models in deep learning. With the knowledge of functions, you can create modular, reusable, and maintainable code that can be easily understood and debugged.
コメント