Haruki Robotics Lab 1 Aug 2023

Magic squares have fascinated humans for thousands of years. A magic square is a grid of numbers, usually positive integers, arranged in such a way that the sum of the numbers in any row, any column, or any main diagonal is always the same constant. This constant is often referred to as the "magic constant".
The simplest magic square is the 1x1 magic square, consisting of a single cell containing the number 1. The next simplest, and the smallest nontrivial case, is the 3x3 square.
The Formula for a Magic Square
In a standard magic square of order `n` (an `n`x`n` square), the magic constant can be calculated using the formula:
`M = n*(n²+1)/2`
where `n` is the size of one side of the square, and `M` is the magic constant.
For example, in a 3x3 magic square, the magic constant M would be `3*((3*3)+1)/2 = 15`.
Generating a Magic Square
There are various methods to construct a magic square. For the case of odd integers, a popular method is the Siamese method (or De la Loubère method). The method follows these steps:
1. Start in the middle of the top row, write 1.
2. Move up and to the right, wrapping around the edges, and write the next number.
3. If the current cell is filled, move down one cell and continue.
4. Repeat the steps until all the cells are filled.
For example, a 3x3 magic square generated using the Siamese method would look like this:
8 1 6
3 5 7
4 9 2
The sums of rows, columns, and diagonals are all 15, the magic constant for this square.
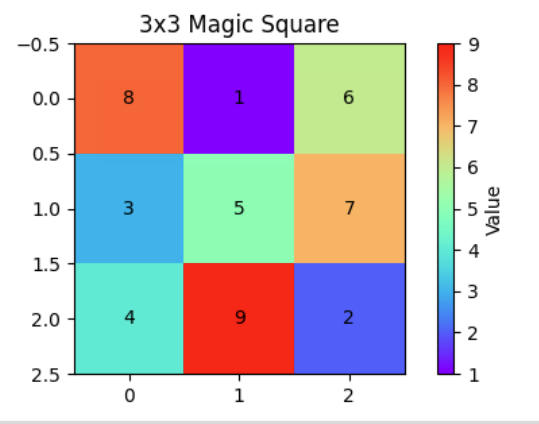
Code
import numpy as np
import matplotlib.pyplot as plt
def generate_magic_square(n):
magic_square = np.zeros((n,n), dtype=int)
nsqr = n*n
i = 0
j = n//2
for k in range(1, nsqr+1):
magic_square[i, j] = k
i -= 1
j += 1
if k % n == 0:
i += 2
j -= 1
elif j==n:
j -= n
elif i<0:
i += n
return magic_square
# Generate a 3x3 magic square
magic_square = generate_magic_square(3)
# Display the magic square as an image
plt.imshow(magic_square, cmap='rainbow')
plt.colorbar(label='Value')
plt.title('3x3 Magic Square')
# Add the numbers to each cell
for i in range(magic_square.shape[0]):
for j in range(magic_square.shape[1]):
plt.text(j, i, magic_square[i, j], ha='center', va='center', color='black')
plt.show()
Comments