We are going to use Makecode & Micropython to program a Micro:bit based smart car in this tutorial. Firstly, we will write a code to achieve certain functions. One program one function, that is. The reason behind is Micro:bit only runs one program at a time. New program will erase the old one once updated. However, there is a way around this. We are to combine as many functions as we can in one single program. Without further ado, let's dive in.
You will need to include the following two packages in the begining of your code. Here are the links:
IR Package: https://github.com/YahboomTechnology/Yahboom_IR
Mbit Car Package: https://github.com/lzty634158/yahboom_mbit_en
If you're using Micropython programming langauge, you need to install the "buildingbit library".
Click here to download the hex file. Choose the right file according to which BBC Micro:bit verison you're using. Afer downloading the hex file, save it to your Micro:bit folder before you start coding.
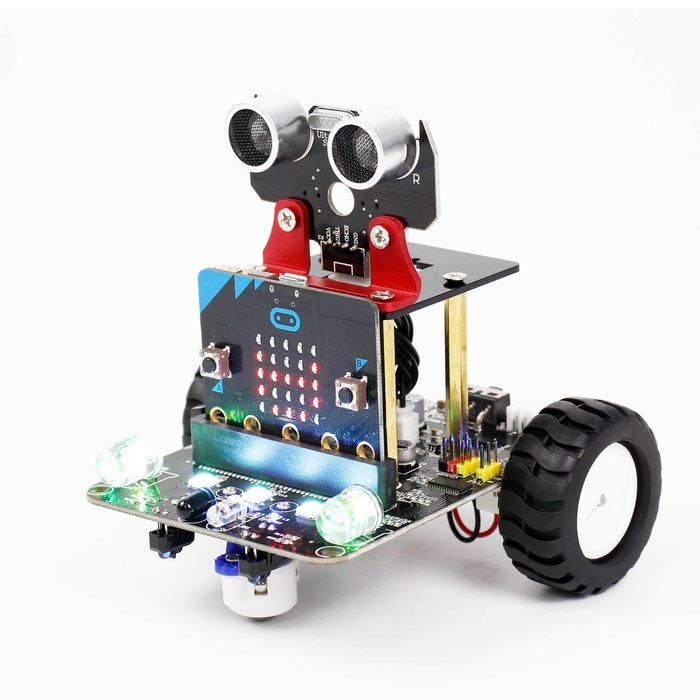
Function 1:
Write a program to move the car forward at an accelerating speed. It gradually speeds up first and gradually decreases back to 0 once hits maximum speed of 225.
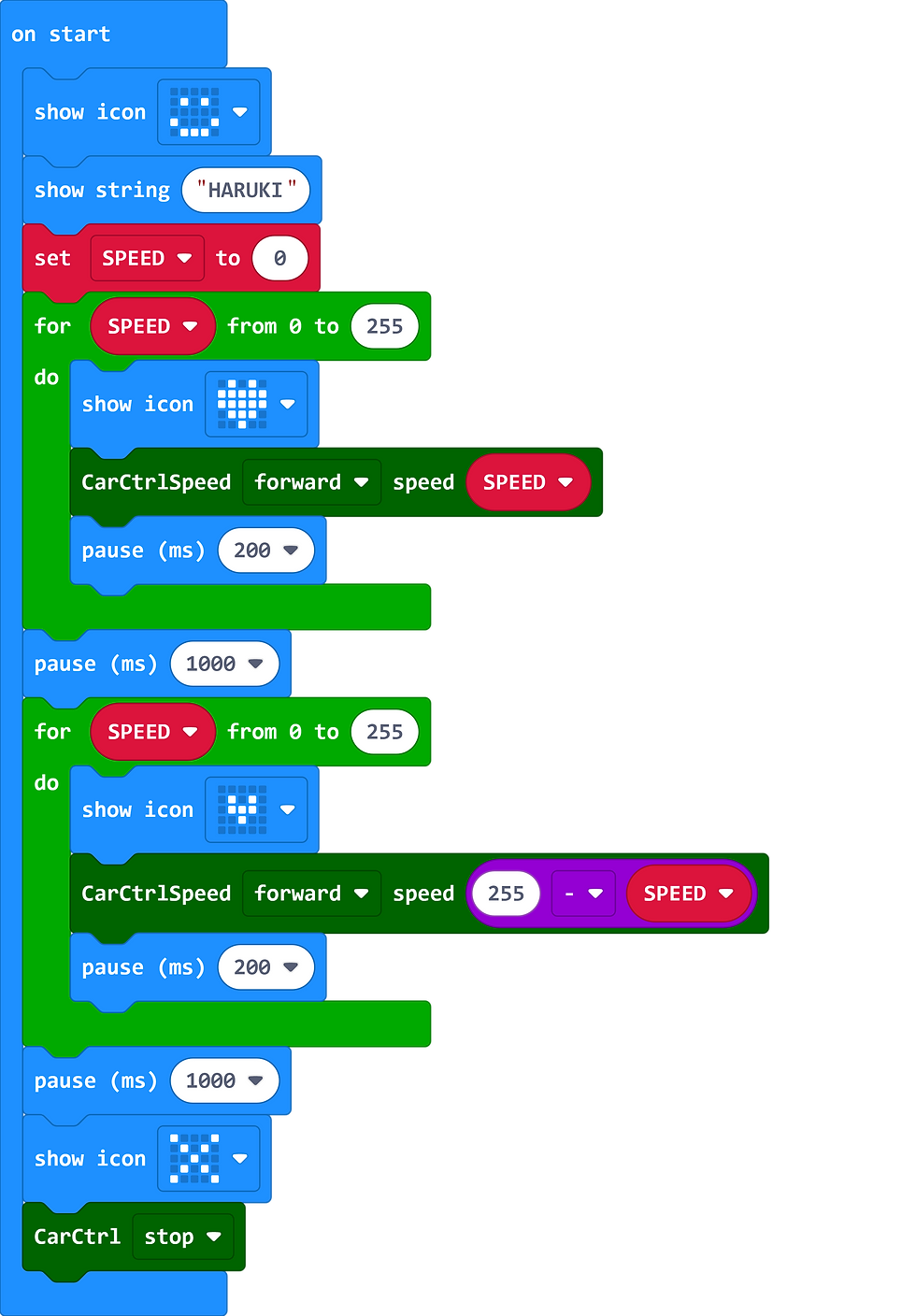
Micropython:
from microbit import*
import buildingbit
speed = 0
#accelerate
for speed in range(0,255,25):
display.show(Image.HEART)
buildingbit.car_run(speed,speed,0)
sleep(200)
#decelerate
for speed in range(0,255,25):
display.show(Image.HEART_SMALL)
buildingbit.car_run(255-speed,255-speed,0)
sleep(200)
Function 2:
Write a program to make the car follow lines on the floor. Since there are built-in Line Tracking Sensors, which can tell black from white, we are easily take advantage of them and add cool feature to your car. Make sure don't set the speed to high here. Here's the code.
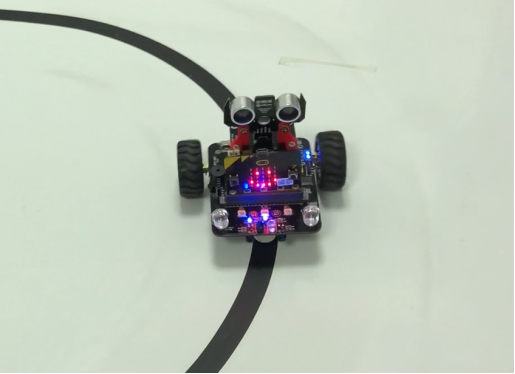
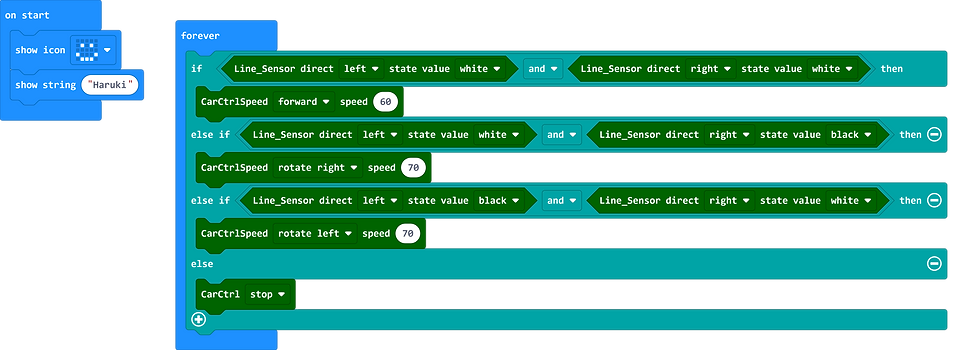
Micropython
#False: white, True: black
from microbit import display, Image
import buildingbit
import random
display.show(Image.HAPPY)
while True:
R = random.randint(0,255)
G = random.randint(0,255)
B = random.randint(0,255)
buildingbit.car_HeadRGB(R,G,B)
sensorL = buildingbit.traking_sensor_L() #true/false
sensorR = buildingbit.traking_sensor_R()
if sensorL is False and sensorR is False:
buildingbit.car_run(80,80,0)
elif sensorL is False and sensorR is True:
buildingbit.car_spinright(80,80,0)
#buildingbit.car_right(80,0)
elif sensorL is True and sensorR is False::
buildingbit.car_spinleft(80,80,0)
else:
buildingbit.car_stop()
display.show(Image.NO)
Function 3:
Write a program to make the car avoid obstacles. We will make full use of the built-in ultrasonic sensor, which is also a distance sensor, to make your car go around when sensing something in front of it.
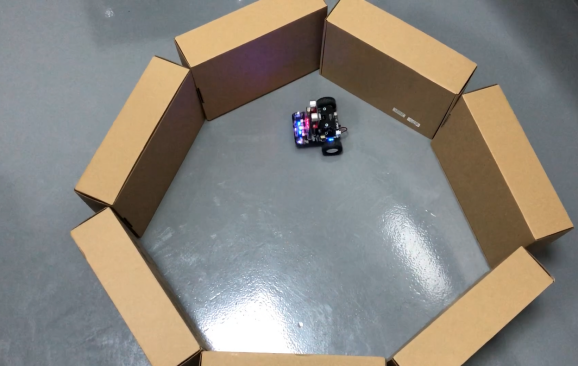

Micropython
from microbit import*
import buildingbit
import music
import random
while True:
distance = buildingbit.ultrasonic()
turn = random.randint(0, 1)
if distance < 20:
buildingbit.car_back(100,100,0)
music.play(music.BA_DING)
sleep(500)
if turn == 0:
buildingbit.car_left(100,0)
display.show(Image.ARROW_W)
sleep(500)
else:
buildingbit.car_right(100,0)
display.show(Image.ARROW_E)
sleep(500)
else:
buildingbit.car_run(80,80,0)
display.show(Image.ARROW_N)
Function 4:
IR Control. There is a built-in IR receiver on the left hand side of the board. It gets signal from a transmitter, your IR Remote. Every time you press a button on the remote, it sends different signal to the car. Hence, we can use those signals as a trigger to whatever actions you want your robot to perfrom. Warning: make sure you are using Verson 1 of the microbit for the program.
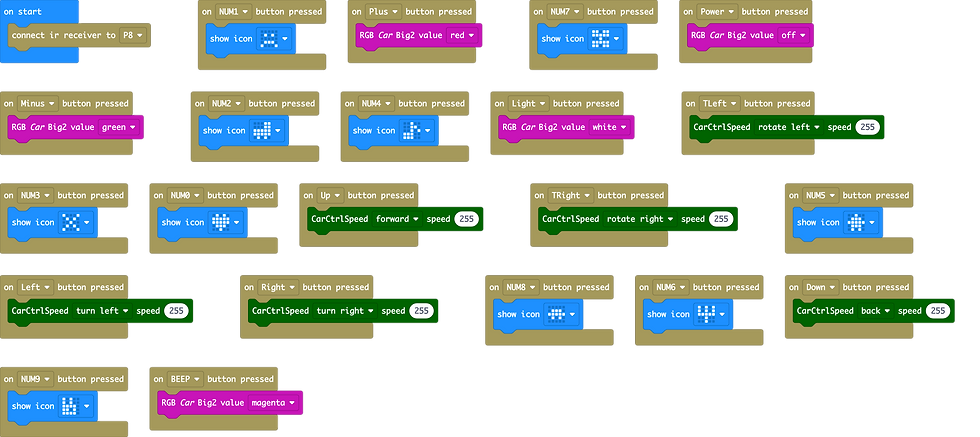
Micropython
from microbit import*
import buildingbit
buildingbit.init_IR(pin8)
buildingbit.car_HeadRGB(0,0,0)
#UP ARROW: 32895
#LEFT ARROW:8415
#RIGHT ARROW: 24735
#DOWN ARROW: 36975
#ON: 255
#VOL: 4105
#1:2295
#2:34935
#3:18615
#4:10455
if key == 32895:
buildingbit.car_run(100,100,0)
elif key == 8415:
buildingbit.car_left(100,0)
elif key == 24735:
buildingbit.car_right(100,0)
elif key == 36975:
buildingbit.car_back(100,100,0)
elif key == 20655:
buildingbit.car_stop()
buildingbit.car_HeadRGB(255,0,0)
sleep(500)
Function 5:
In this program we'll include line tracking and avoidance functions all into one.
This is the code:
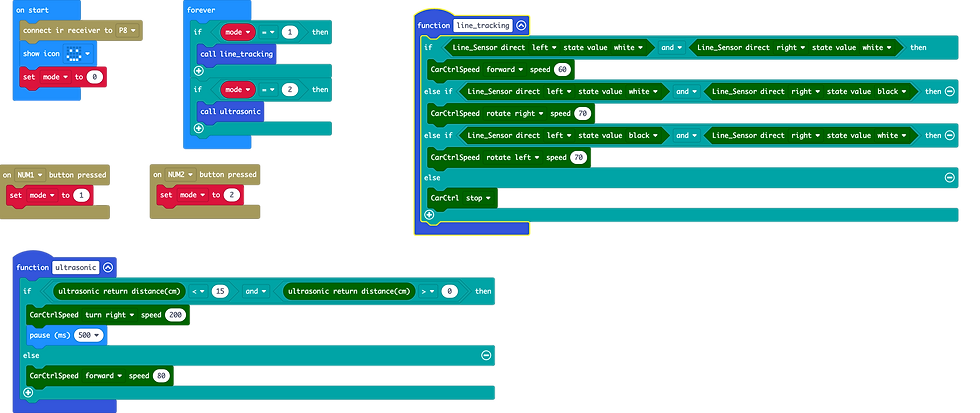
Comments