JavaScript is a powerful, flexible, and popular programming language that is primarily used for building interactive elements on websites. It's one of the three core technologies of web development, along with HTML and CSS. This post will guide students from Level 1 (Beginner) to Level 3 (Intermediate).
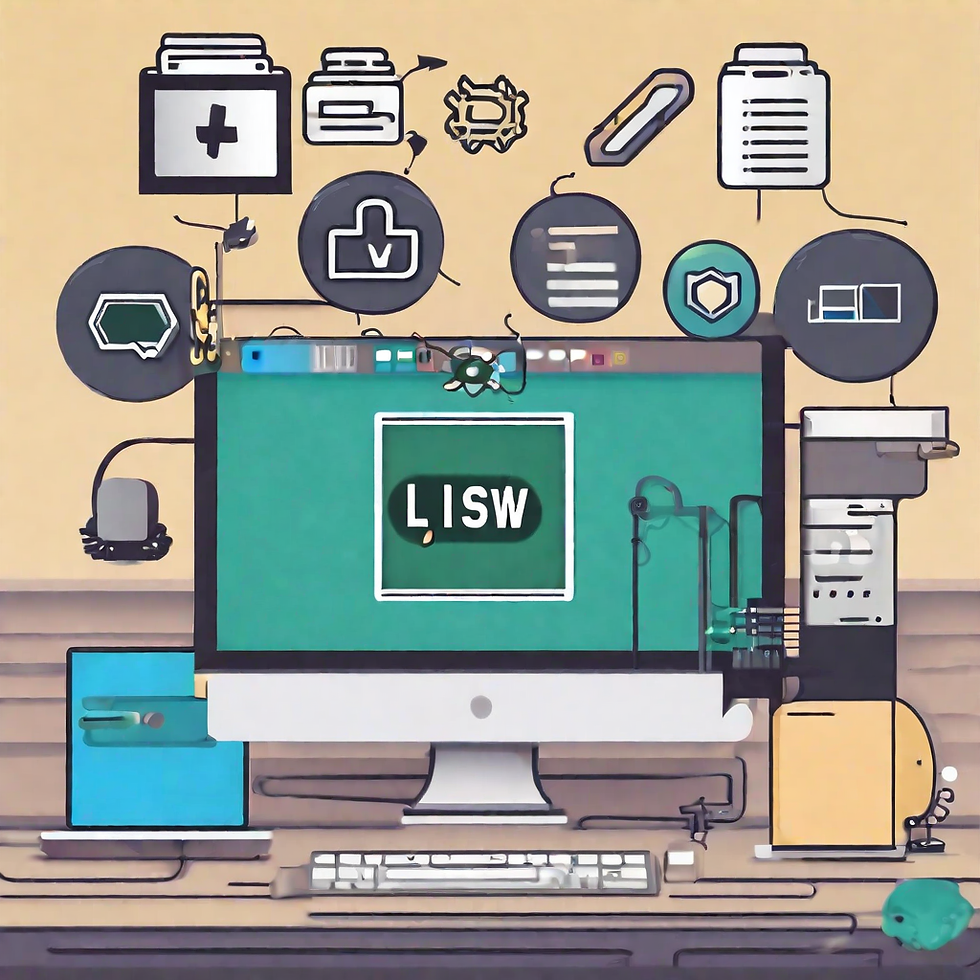
Level 1: The Basics
What is JavaScript?
JavaScript was initially created to make web pages more interactive. It is a high-level, interpreted language that allows you to implement complex features on web pages. These features can include displaying timely content updates, interactive maps, animated 2D/3D graphics, scrolling video jukeboxes, and more!
Including JavaScript in Your HTML
To include JavaScript in your HTML, you can write it directly within script tags in the HTML file, or link to an external JavaScript file.
Here's an example of JavaScript in an HTML file:
<!DOCTYPE html>
<html>
<body>
<h2>My First JavaScript Program</h2>
<button type="button"
onclick="document.getElementById('demo').innerHTML = Date()">
Click me to display Date and Time.</button>
<p id="demo"></p>
</body>
</html>
In the above snippet, when you click the button, the JavaScript function is triggered to display the current date and time.
Level 2: Variables, Functions, and Control Structures
Variables
Variables in JavaScript are containers for storing data values. In JavaScript, you can declare a variable using the `var`, `let`, or `const` keyword. Here's an example:
let name = "John";
const pi = 3.14159;
Functions
Functions are blocks of code designed to perform a particular task. Here is a simple JavaScript function:
function sayHello() {
alert("Hello, World!");
}
You can then "call" or "invoke" this function like this:
sayHello();
Control Structures
Control structures like `if`, `for`, and `while` can control the flow of the program. Here's an example of an `if` statement:
let x = 10;
if (x > 5) {
alert("x is greater than 5");
}
Level 3: Working with the DOM and Events
The Document Object Model (DOM)
The DOM is a programming interface for web documents. It represents the structure of a document and allows a programming language like JavaScript to manipulate the document's structure, style, and content. Here's an example:
document.getElementById("myHeader").innerHTML = "Hello, World!";
Events
JavaScript can also respond to user interactions through events. An event can be something the browser does, or something a user does. Here's an example:
document.getElementById("myButton").onclick = function() {
alert("You clicked the button!");
};
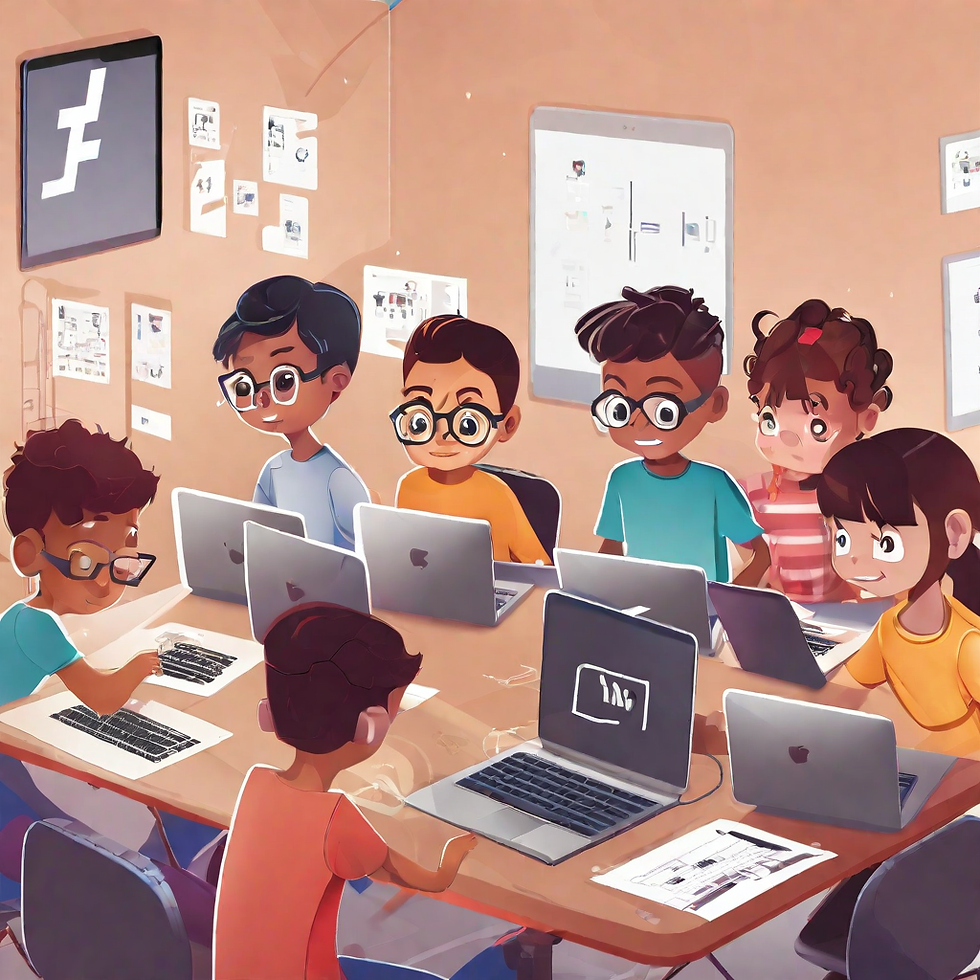
Exercises
Level 1: The Basics
1. Create an HTML page with a button. When the button is clicked, display a pop-up alert with the message "Hello, World!"
Level 2: Variables, Functions, and Control Structures
2. Declare a variable `x` with the value `10` and a variable `y` with the value `20`. Write a function `addNumbers` that returns the sum of `x` and `y`. Log the result to the console.
3. Using a `for` loop, log numbers 1 through 10 to the console.
Level 3: Working with the DOM and Events
4. Create an HTML page with a paragraph with the id "myPara". Use JavaScript to change the text of that paragraph to "JavaScript is fun!"
5. Create an HTML page with a button. When the button is clicked, change the color of the page background to blue.
Answers
Level 1: The Basics
1. Create an HTML page with a button. When the button is clicked, display a pop-up alert with the message "Hello, World!"
<!DOCTYPE html>
<html>
<body>
<button onclick="alert('Hello, World!')">Click me!</button>
</body>
</html>
Level 2: Variables, Functions, and Control Structures
2. Declare a variable `x` with the value `10` and a variable `y` with the value `20`. Write a function `addNumbers` that returns the sum of `x` and `y`. Log the result to the console.
let x = 10;
let y = 20;
function addNumbers(a, b) {
return a + b;
}
console.log(addNumbers(x, y)); // Outputs: 30
3. Using a `for` loop, log numbers 1 through 10 to the console.
for (let i = 1; i <= 10; i++) {
console.log(i);
}
Level 3: Working with the DOM and Events
4. Create an HTML page with a paragraph with the id "myPara". Use JavaScript to change the text of that paragraph to "JavaScript is fun!"
<!DOCTYPE html>
<html>
<body>
<p id="myPara">Hello, World!</p>
<script>
document.getElementById("myPara").innerHTML = "JavaScript is fun!";
</script>
</body>
</html>
```
5. Create an HTML page with a button. When the button is clicked, change the color of the page background to blue.
<!DOCTYPE html>
<html>
<body>
<button onclick="changeBackground()">Click me!</button>
<script>
function changeBackground() {
document.body.style.backgroundColor = "blue";
}
</script>
</body>
</html>
Comments